Working with Vue.js templates
We have already walked through the basics of using templates in Vue.js, where we saw how to use string interpolation to output data. I want to expand a bit on that and show you that you can also use simple JavaScript expressions within the string interpolation syntax. The reason I said simple JavaScript expressions is that you can only include a single expression. Therefore you cannot use loops or any complex logic. This is okay anyways, as such logic would not belong in our template anyways; it would be better off being placed within a method in our Vue instance – something that I will get back to in the next article.
For now, let me just show you a simple example. Suppose I have an age property within my data object which contains an arbitrary number.
For whatever reason, I want to output this number multiplied by two. I can do so by adding a simple JavaScript expression directly in my template within the string interpolation syntax.
<div id="app">
<h1>{{ age * 2 }}</h1>
</div>
Here I just multiply the age property by two, and if I run the code, we should see the expected number being output. Remember that I could access the age property directly within my template because Vue.js proxies the data properties for me, so I did not have to access the property on the data object explicitly. In fact, if you tried to do so, it would not work.
Let’s take a look at how we can use boolean logic within our template. Since you can only use a single expression, you cannot use normal if statements. However, what you can do, is to use shorthand if statements. Suppose that I want to output whether a person is old or young. I could check if the age is greater than a certain number and if so, output an arbitrary string.
<div id="app">
<h1>{{ age > 60 ? 'Old' : 'Young' }}</h1>
</div>
In this case, I will check if the age property contains a number that is greater than 60. In that case, I will output “Old” – otherwise, “Young” will be displayed. If I run the code, it luckily determines that I am still young, which is pretty fortunate for me! If I change the age property to 80, it will output “Old” instead.
new Vue({
el: '#app',
data: {
age: 80
}
});
Let’s see one more example of an expression. I will add my full name to the data property as the name property.
new Vue({
el: '#app',
data: {
age: 27,
name: 'Bo Andersen'
}
});
The value includes both my first and last name, but I only want to display my first name on the page. What I can do is to split the name by spaces.
<div id="app">
<h1>{{ name.split(' ') }}</h1>
</div>
If I run this, an array of the two elements will be output. To output my first name, I can output the element at index zero, like this.
<div id="app">
<h1>{{ name.split(' ')[0] }}</h1>
</div>
If I run the code now, you will see my first name being output.
This was just another example of an expression. You can do a lot of things with a single JavaScript expression, but just try to keep it simple. If you need more complex logic, then you should not try to force it into the template, such as nested shorthand if statements. Also, if you need to use the same expression multiple times in your template, then it would probably be better to not embed it within the template itself, but within the Vue instance instead. This can be done with methods, which we will take a look at now.
Here is what you will learn:
- How to build advanced Vue.js applications (including SPA)
- How Vue.js works under the hood
- Communicating with services through HTTP
- Managing state of large applications with Vuex
- ... and much more!
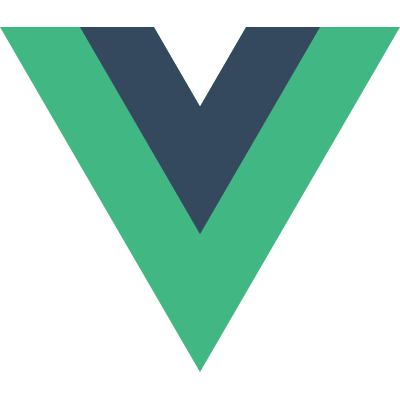